How to Use Web Services in ASP.NET Client Side:-
For using of Web Services in ASP.NET Client follow the following steps:-
Step(1):-
Create New ASP.NET Application named is CalculatorWebAppClient as shown in below.
Step 2:- Now we need to add a reference to web service.
To achieve this:-
a) Right click on References folder in the CalculatorWebAppClient project and select Add Service Reference option.
b) In the Address textbox of the Add Service Reference window, type the web service address and click GO button. In the namespace textbox type CalculatorWebServices and click OK as shown in below image.
Step 3:- Right click on CalculatorWebAppClient project in solution explorer and add new webform.
Step 4:- Copy and paste the following HTML code.
Step 5- Copy and paste the following code in the code-behind file
Step(6):-Now, run the application and get output as expected.
Step(1):-
Create New ASP.NET Application named is CalculatorWebAppClient as shown in below.
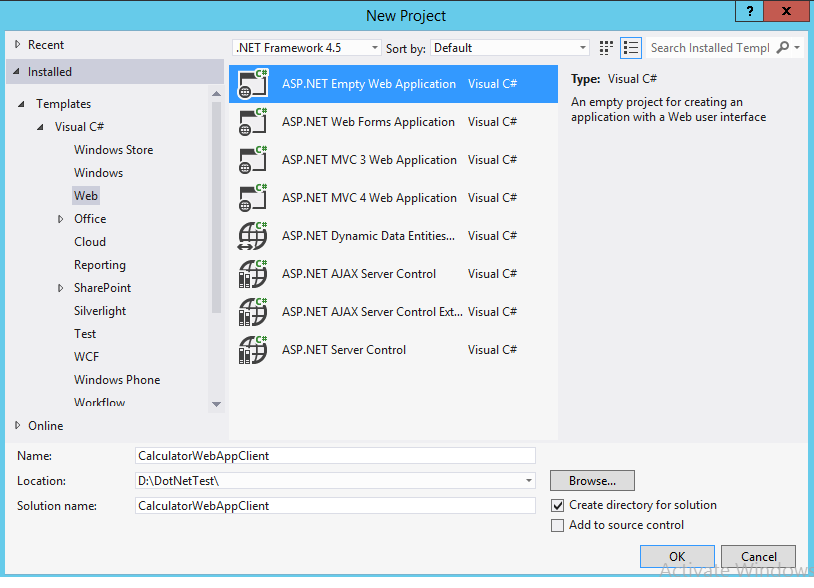
Step 2:- Now we need to add a reference to web service.
To achieve this:-
a) Right click on References folder in the CalculatorWebAppClient project and select Add Service Reference option.
b) In the Address textbox of the Add Service Reference window, type the web service address and click GO button. In the namespace textbox type CalculatorWebServices and click OK as shown in below image.
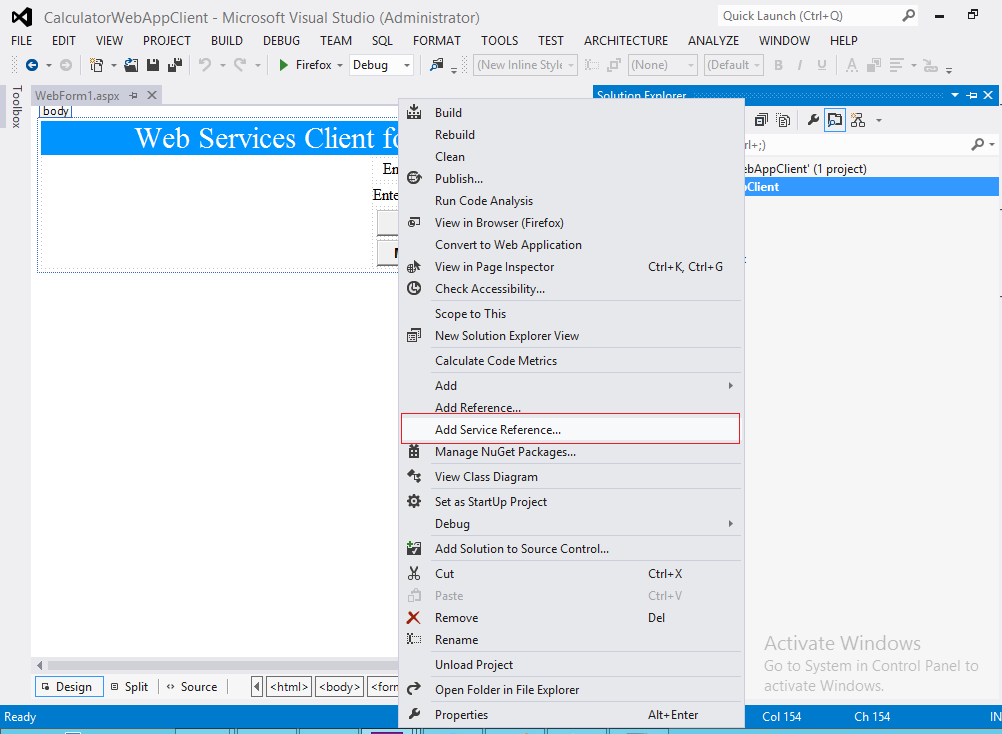
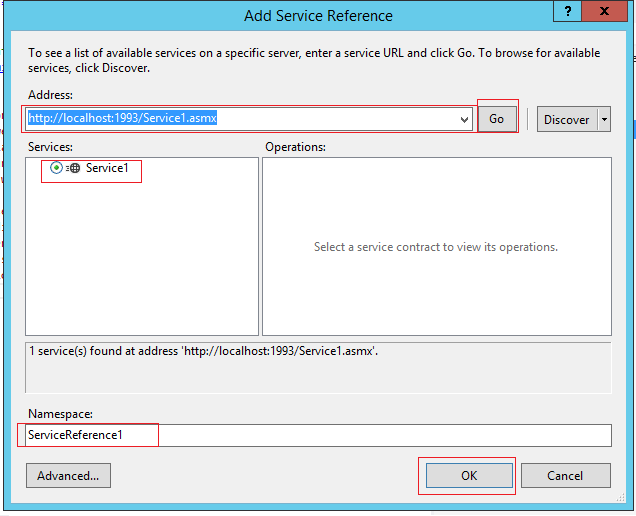
Step 3:- Right click on CalculatorWebAppClient project in solution explorer and add new webform.
Step 4:- Copy and paste the following HTML code.
Step 5- Copy and paste the following code in the code-behind file
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; namespace CalculatorWebAppClient { public partial class WebForm1 : System.Web.UI.Page { //create the object of Service ServiceReference1.Service1SoapClient objService = new ServiceReference1.Service1SoapClient(); protected void Page_Load(object sender, EventArgs e) { } //addtion code protected void btnAddtion_Click(object sender, EventArgs e) { int firstno = Convert.ToInt32(txtFirstNo.Text); int secondno = Convert.ToInt32(txtSecondNo.Text); int add= objService.Addition(firstno,secondno); lblMessage.Text ="The addition of two number "+ add.ToString(); } //subtration protected void btnSubtration_Click(object sender, EventArgs e) { int firstno = Convert.ToInt32(txtFirstNo.Text); int secondno = Convert.ToInt32(txtSecondNo.Text); int sub = objService.Subtration(firstno, secondno); lblMessage.Text ="The subtration of two number "+ sub.ToString(); } //multiplication protected void btnMultiplication_Click(object sender, EventArgs e) { int firstno = Convert.ToInt32(txtFirstNo.Text); int secondno = Convert.ToInt32(txtSecondNo.Text); int mul = objService.Multiplication(firstno, secondno); lblMessage.Text ="The multiplication of two number "+ mul.ToString(); } //division protected void btnDivision_Click(object sender, EventArgs e) { int firstno = Convert.ToInt32(txtFirstNo.Text); int secondno = Convert.ToInt32(txtSecondNo.Text); int div = objService.Devision(firstno, secondno); lblMessage.Text ="The division of two number "+ div.ToString(); } } }
Step(6):-Now, run the application and get output as expected.
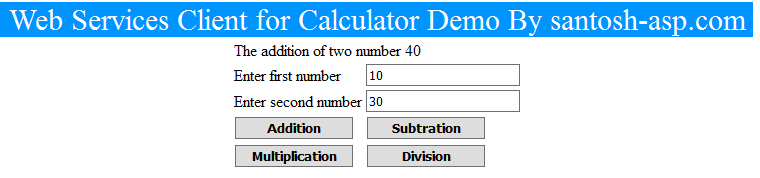